Transmission Control Protocol(TCP) is the prevalent communication standard for the Internet. Every email sent and every webpage loaded travels from server to client as TCP data packets with the promise of reliable delivery. However, while ensuring order and integrity, TCP also creates some inefficiencies, particularly in speed and latency. You may barely notice this in day-to-day browsing, yet they significantly impact the performance of real-time, high-bandwidth applications. Issues such as slow start, head-of-line blocking, and time-consuming handshakes are prominent.
Quick UDP Internet Connections(QUIC) is an upcoming protocol that addresses TCP performance issues by enhancing efficiency and providing a more adaptable and secure foundation for digital communications. It operates on top of User Datagram Protocol (UDP), which requires no handshakes and minimizes overheads for efficiency.
This article explores how QUIC builds on and diverges from TCP's legacy and its practical impacts on internet performance. We also provide monitoring tips to ensure QUIC vs. TCP gives you the needed performance improvement.
As we dive into technical details from the start, it is a good idea to review the basics from Chapter 1 of this guide before getting started.
QUIC vs. TCP—Summary of key differences
The rest of this article explores these differences in detail.
QUIC vs. TCP differences in protocol implementation
QUIC and TCP are designed for reliable data transfer but have distinct differences in their design and implementation. Both ensure reliable data delivery and manage congestion while fundamentally providing data transmission across networks. However, there are places where both have differences.
- QUIC enables 0-RTT (zero round trip time) for faster connection establishment, while TCP requires a three-way handshake which adds latency.
- QUIC supports multiple independent streams within a single connection, reducing head-of-line blocking, while TCP handles streams sequentially.
- QUIC uses explicit packet numbering for all transmitted packets, so ACKs specify which packets have been received. TCP ACK implementation creates ambiguity as it can be unclear whether an ACK is for new or retransmitted data.
- QUIC prevents unnecessary slowdowns by identifying between actual congestion and transient network delay. Traditional slow-start methods in TCP can lead to premature reductions in the congestion window due to misinterpreted congestion signals.
QUIC generally outperforms TCP, except for the following exceptions.
- QUIC is sensitive to out-of-order packet delivery, interpreting such behavior as loss, and performing significantly worse than TCP.
- QUIC performance diminishes on mobiles due to resource contention, while kernel-implemented TCP handles packet processing more efficiently on resource-constrained devices.
{{banner-6="/design/banners"}}
Flexibility
As a protocol implemented in the kernel space, any significant modifications to TCP require changes at the kernel level. This can involve extensive development cycles, including kernel recompilation, system reboots, and comprehensive testing to ensure stability across different environments.
Implementing protocols in user space, as QUIC does, allows developers to update and iterate on their software without modifying the operating system kernel. Changes can be rolled out faster because they don't require kernel version updates or system reboots. Errors or security vulnerabilities within a user-space application are less likely to compromise the entire operating system. User-space implementations can also dynamically allocate and manage resources such as memory and CPU without interfering with the kernel’s management of critical system resources.
Below is the example code snippet of QUIC implementation written in Rust using the “quiche” library:
//Configuring connections
let mut config = quiche::Config::new(quiche::PROTOCOL_VERSION)?;
config.set_application_protos(&[b"example-proto"]);
// Client connection.
let conn =
quiche::connect(Some(&server_name), &scid, local, peer, &mut config)?;
// Server connection.
let conn = quiche::accept(&scid, None, local, peer, &mut config)?;
//handling incoming connections
let to = socket.local_addr().unwrap();
loop {
let (read, from) = socket.recv_from(&mut buf).unwrap();
let recv_info = quiche::RecvInfo { from, to };
let read = match conn.recv(&mut buf[..read], recv_info) {
Ok(v) => v,
Err(quiche::Error::Done) => {
// Done reading.
break;
},
Err(e) => {
// An error occurred, handle it.
break;
},
};
}
The above code initiates the connection to the server, accepts a connection from a client, handles incoming data using the connection object, and later processes the packets. For more detailed code examples, please visit this documentation.
{{banner-9="/design/banners"}}
Server-side configuration reuse
Every time a client reconnects using TCP, it must go through a complete handshake process. This involves verifying the client’s credentials and re-establishing network parameters, which introduces delays. TCP has no built-in mechanism to store or reuse server-side configurations for subsequent connections.
QUIC enhances efficiency through server-side configuration reuse. When a client first connects to a server, QUIC allows the server to store specific configuration details, such as cryptographic parameters and connection settings. This stored information can then be quickly reused for future connections from the same client, bypassing the need for a complete handshake each time and improving application responsiveness.
The below function sends data to the server before the connection is fully established on subsequent connections:
config.set_enable_early_data(true);
Please visit QUIC docs for more details on the parameters of the above function and return type.
Acknowledgement ranges
TCP generally requires that packets be acknowledged in the order they are received. Selective Acknowledgement (SACK), specified in RFC 2018, is an optional feature that allows TCP to acknowledge non-contiguous blocks. For example, if TCP sends packets 1, 2, 3, and 4, and packet 3 is lost or delayed, SACK allows TCP to acknowledge packets 1, 2, and 4, enabling packet 3 to be retransmitted. However, SACK must be supported by both the sender and receiver.
QUIC has selective acknowledgment built in. Its approach to congestion control is different from TCP's. When detecting packet loss, TCP often reduces its congestion window (the amount of data it can send before needing an acknowledgment). In contrast, QUIC adjusts its congestion control more granularly to maintain higher throughput.
QUIC also maintains detailed state information for each packet, which allows it to adjust its congestion window incrementally, avoiding severe reductions and maintaining smoother performance. It always uses a hybrid slow start algorithm, which dynamically adjusts the window size based on real-time monitoring of round-trip times. It prevents unnecessary slowdowns by identifying between actual congestion and transient network delay. Traditional slow-start methods in TCP can lead to premature reductions in the congestion window due to misinterpreted congestion signals.
{{banner-3="/design/banners"}}
Cryptographic agility
QUIC treats each data stream independently. For enhanced security measures, separate encryption keys are used for each stream. It uses TLS 1.3 for its encryption, which is built into the protocol rather than a separate layer. In our separate chapter, you can learn all about the advantages TLS 1.3 brings compared to older protocols.
QUIC also uses source-address tokens, unique cryptographic tokens created based on previous client and server interactions. When a client makes a new connection request, it includes one of these tokens so the server can verify that the request is coming from a known and previously verified source rather than malicious traffic. These tokens offer a robust way to prevent replay attacks, where an attacker copies a valid data transmission and replays it to create unauthorized injection attacks, where malicious data is inserted into legitimate traffic.
In contrast, TCP itself does not provide encryption; it relies on TLS to secure connections. TLS over TCP requires a complete handshake to establish the TCP connection before a second handshake for the TLS encryption, increasing the initial setup time. While effective for encrypting data and ensuring the integrity of communication, it does not prevent injection attacks without integrating additional protocols.
Monitoring tips
While QUIC and TCP differ in design and implementation, you can monitor both using the same tools and methods. Network administrators can accurately assess the impact on application performance and user experience by measuring both protocols with consistent metrics under similar conditions. We give some common metrics below.
QUIC vs. TCP multipath operations
Multipath means the ability to use multiple network paths to transmit data between a collection of IP addresses from a given source and a single destination using multiple paths. TCP can send packets through various routes, but it treats each path as a separate session. However, Multipath TCP (MPTCP) can combine multiple source addresses into a single session, allowing for concurrent use of several paths. This means data packets can travel through different routes simultaneously, enhancing overall connection reliability and performance
Multipath QUIC can also utilize various network paths between the same client and server. For example, a mobile device could use Wi-Fi and cellular networks to maintain a single QUIC session. It aims to provide the best possible performance and minimize latency. QUIC multipath ability also increases the total bandwidth available and provides redundancy, ensuring that the failure of one path doesn't disrupt the connection.
For example, in Google's deployment of QUIC for global load balancing, QUIC optimizes data flows based on real-time network conditions. It adjusts traffic routes dynamically to avoid congestion and reduce packet loss rates. Similarly, Facebook uses QUIC to enhance the delivery of live video content for uninterrupted streaming, as it can switch to the most efficient available path without dropping the connection.
TCP is natively confined to a single path, limiting its ability to dynamically handle network transitions and congestion. However, Multipath TCP (MPTCP), an extension of TCP, enables multiple paths to be used simultaneously by a single TCP connection, enhancing bandwidth utilization and providing redundancy. However, MPTCP isn't part of standard TCP and requires additional setup and support from both the client and server.
{{banner-23="/design/banners"}}
Monitoring tips
Is QUIC meeting your multipath operation requirements?
- Monitor throughput and latency on each path using the formula total data transferred / total time. Having these metrics helps identify the best-performing paths, allowing smarter resource allocation and path selection
- Analyze performance before and after path adjustments during peak times with time difference. It provides insights into how network adjustments affect real-time data flow.
- Track uptime and reliability across different network conditions.
By monitoring these metrics closely, you can ensure your network remains resilient and responsive and delivers high performance under all conditions.
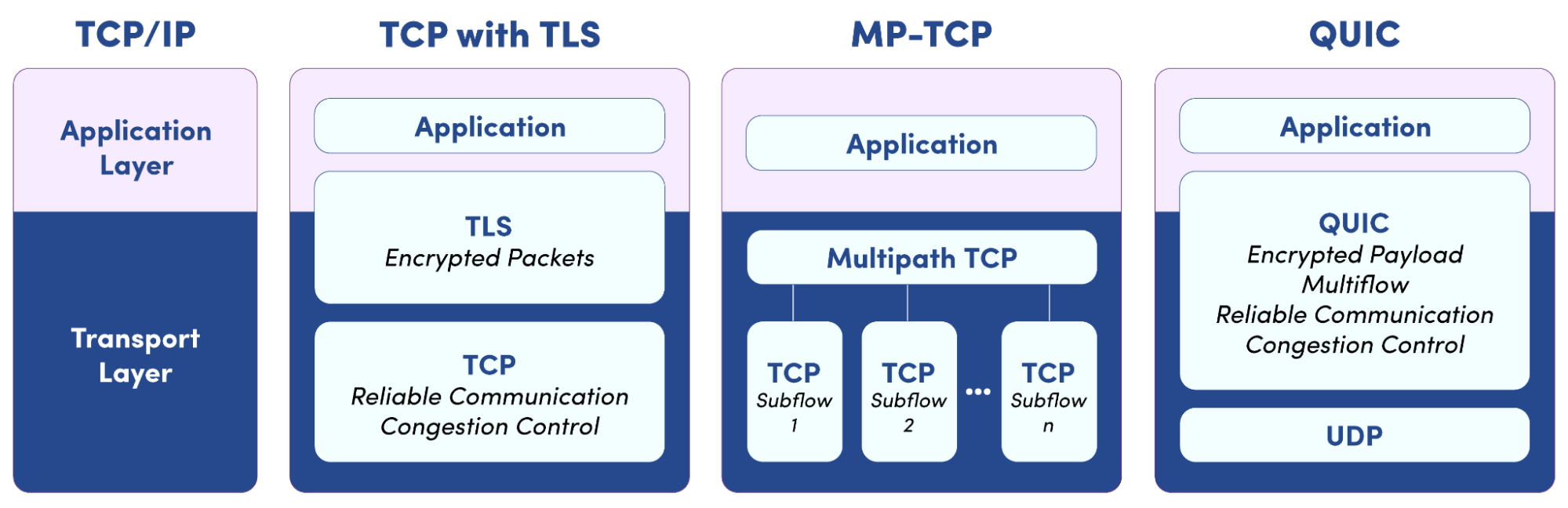
QUIC vs. TCP connection migration
Connection migration allows a network connection to continue seamlessly even if the underlying path changes. This is crucial for maintaining uninterrupted service when a device switches networks such as from Wi-Fi to cellular.
Connection migration in QUIC has a unique identifier for a conversation that both the client and server recognize, regardless of how the message is delivered. When a QUIC connection is established, a set of Connection IDs is negotiated and can be used interchangeably.
TCP connections are identified and handled by the 5-tuples of client IP, client port, server IP, server port, and protocol. If any of these change, the connection must be re-established. QUIC instead creates a Connection ID, which can be sent over different connections. You can reuse the settings without starting a connection from scratch. The client and server negotiate a set of Connection IDs when the connection is initiated.
Simply put, QUIC connection migration saves you the effort of setting up a reliable and secure connection. Traditional TCP requires a three-way handshake. It takes time and round trips, resulting in a slow start. With QUIC, you benefit from not having to do the expensive initial 3-way TCP handshake and then the even more expensive TLS handshake.

Role of IP in QUIC
QUIC still uses IP underneath. So, you need an IP address to send and receive messages back. When using QUIC, if you initiate a connection from one IP address (IP address 1 using Wi-Fi), you expect responses to return to that same IP address. However, switching to a different network, such as moving from Wi-Fi to a mobile data network (changing to IP address 2), might initially miss some responses due to this change.
QUIC handles this scenario using stable connection IDs. Even when your IP address changes, the connection ID remains the same. Therefore, the server recognizes the existing connection ID the next time you send a message, even from the new IP address. It understands that some packets were likely lost during the transition and resends them accordingly.
For instance, given Uber's mobile nature, maintaining stable and continuous connections during network transitions (e.g., switching from Wi-Fi to cellular data) is crucial for drivers and riders. QUIC connection migration capabilities have been integral to achieving this seamless connectivity. To learn more, please visit.
Monitoring tips
Assessing the effectiveness of QUIC connection migration using specific metrics that reflect its impact on user experience and network stability:
When to use QUIC vs. TCP
QUIC can be used for applications where low latency and improved packet loss handling are critical, including environments with high round-trip time (RTT) and lossy networks. QUIC significantly reduces connection and reconnection times for services requiring secure and immediate data transfer, such as video conferencing and online gaming,
It is also ideal for web applications that load multiple resources simultaneously, such as media-rich websites. Its ability to handle various data streams independently helps ensure that a delay in one stream (due to packet loss) does not affect the loading of other resources.
QUIC is beneficial if your connection is unstable, as it supports connection migration. This means that if a user's IP address changes, the connection can continue without needing to be re-established.
{{banner-15="/design/banners"}}
TCP use cases
Although QUIC capabilities are advantageous, TCP is also undergoing enhancements. Efforts like TCP Fast Open and improvements in congestion control algorithms (such as BBR) show that TCP is still very much a protocol in development, adapting to new network demands and maintaining its relevance alongside QUIC.
TCP’s established reliability remains a preferred protocol in many use cases. Its wide use and extensive support across almost all platforms and networks make it a safe and stable choice.
The extensive auditing of TCP's behavior under various network conditions makes it a reliable choice for scenarios that prioritize risk management. TCP may also be more appropriate in environments that use hardware offloading, such as TCP segmentation offload and large receive offload.
In scenarios where CPU resources are limited because QUIC user-space processing can require more CPU power, mainly when dealing with high bandwidth. TCP is better for straightforward bulk data transfers across reliable and stable networks.
Conclusion
Choosing the appropriate protocol, QUIC or TCP, can significantly impact your applications' efficiency, security, and reliability. QUIC advances beyond TCP capabilities and excels in environments requiring rapid data transmission, enhanced security, and high resilience. TCP remains a steadfast choice for scenarios demanding broad compatibility and less complex network requirements. By understanding these distinctions and evaluating your applications' specific needs, you can effectively choose the protocol that best aligns with your technical and operational goals.